Get Started - Learn How To Make Your Bot!GuidesCode SnippetsGet methodsPost methodsWeb APIUseful linksList of all currently Free ItemsList of EmotesHighrise Bot SDK Changelog
Concept
Want a easier way to change your bot’s outfit color? You found it!
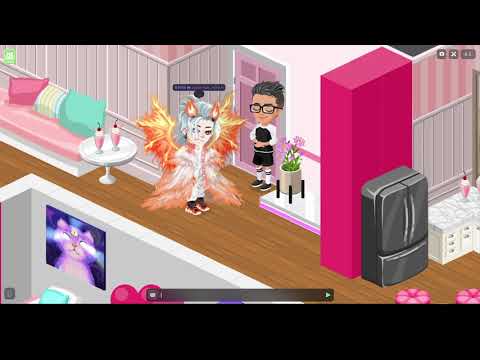
The code that we’re going to use today is very similar to the code at .
Today we are gonna make a function to change thebot’s outfit color using on_chat commands. To do that we will use the 📬 Command Handler, note that this use is optional, you can implement this function directly on your main code or directly on your on_chat method.
The command works by saying the command (color), the item category, and the color number in the Highrise palette:
Categories
blush
body
hair_front
hair_back
eye
face_hair
mouth
Colors
The color palette in Highrise is a number that represents an array position, always starting at 0 and can range to diferent sizes depending on the item category. You can check which number represents each color in your avatar editor by counting the colors starting from 0.
MAKE SURE TO READ HOW CHANGING THE OUTFIT WORKS ABOVE:
Methods/Functions used:
Required Libraries
- highrise-bot-sdk
Code
First of all, let’s create a new file called color.py inside of our functions folder:

Here’s what we will need to import into our color.py file:
from highrise import * from highrise.models import *
Let’s proceed to our function definition, since we’re using the command handler for this example, your function name will be the command that you will use in the chat to call it, I will name mine as color:
from highrise import * from highrise.models import * async def color(self: BaseBot, user: User, message: str):
Finally, let’s implement the function and after it you will see a detailed step by step guide on how the code works:
from highrise import * from highrise.models import * async def color(self: BaseBot, user: User, message: str): parts = message.split(" ") print (parts) if len(parts) != 3: await self.highrise.chat("Invalid command format. You need to specify the category and color palette number.") return category = parts[1] try: color_palette = int(parts[2]) except: await self.highrise.chat("Invalid command format. You need to specify the category and color palette number.") return outfit = (await self.highrise.get_my_outfit()).outfit for outfit_item in outfit: #the category of the item in an outfit can be found by the first string in the id before the "-" character item_category = outfit_item.id.split("-")[0] if item_category == category: try: outfit_item.active_palette = color_palette except: await self.highrise.chat(f"The bot isn't using any item from the category '{category}'.") return await self.highrise.set_outfit(outfit)
Now let’s understand how the code works:
from highrise import *
andfrom highrise.models import *
:- These lines import the necessary modules and classes required for interacting with the game's API.
async def color(self: BaseBot, user: User, message: str):
- This is an asynchronous function called
color
. - It takes four parameters:
self
(a reference to the instance of the class this method belongs to),user
(an object representing the user who sent the chat message), andmessage
(a string representing the chat message sent by the user).
parts = message.split(" ")
:- The function splits the
message
string into individual words using space as the separator and stores them in the listparts
.
print(parts)
:- This line prints the list of words in the
message
to the console. It can be useful for debugging purposes to check what the user's input looks like.
if len(parts) != 3:
:- If there are not exactly three words in the
message
, it means the user has not specified the category and color palette correctly. - The function sends a message to the chat using
self.highrise.chat()
to inform the user about the invalid command format and then returns, ending the function.
category = parts[1]
andcolor_palette = int(parts[2])
:- These lines extract the category (the second word) and the color palette (the third word) from the
parts
list and store them incategory
andcolor_palette
variables, respectively.
(await self.highrise.get_my_outfit()).outfit
:- The code calls an asynchronous method
get_my_outfit()
onself.highrise
to fetch the bot's outfit items. - The result is awaited using
await
. - The method seems to return some kind of response object, and
outfit
appears to be the actual list of items in the bot's outfit.
- The code then iterates through the outfit items to find the items of the specified
category
.
item_category = outfit_item.id.split("-")[0]
:- This line extracts the category of the current
outfit_item
by splitting the item's ID using the "-" character and taking the first part (before the "-").
if item_category == category:
:- If the category of the current
outfit_item
matches the specifiedcategory
, the code tries to set theactive_palette
property of that item to the providedcolor_palette
.
try: outfit_item.active_palette = color_palette
:- The code tries to set the
active_palette
property of theoutfit_item
to the specifiedcolor_palette
.
except: await self.highrise.chat(f"The bot isn't using any item from the category '{category}'.")
:- If the
outfit_item
is not found for the specifiedcategory
, an exception is raised (likely becauseoutfit_item
isNone
or it doesn't have anactive_palette
property). - The code catches this exception and sends a message to the chat using
self.highrise.chat()
to inform the user that the bot isn't using any item from the specified category. - The function then returns, ending the execution.
await self.highrise.set_outfit(outfit)
:- After potentially updating the
active_palette
property for the outfit item(s) of the specifiedcategory
, the code calls an asynchronous methodset_outfit(outfit)
onself.highrise
. - It seems like this method is used to update the bot's outfit using the modified
outfit
list.