Get Started - Learn How To Make Your Bot!GuidesCode SnippetsGet methodsPost methodsWeb APIUseful linksList of all currently Free ItemsList of EmotesHighrise Bot SDK Changelog
Concept
This is a simple code snippet to show how to make a target user perform a given emote using only on_chat commands:
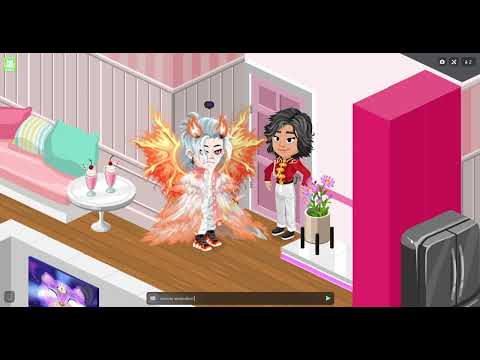
Today we are gonna make a function to make a target user perform a given emote using on_chat commands. To do that we will use the π¬ Command Handler, note that this use is optional, you can implement this function directly on your main code or directly on your on_chat method.
The command format used is
/emote <username> <emote_id>
.Methods/Functions used:
Required Libraries
- highrise-bot-sdk
Code
First of all, letβs create a new file called emote.py inside of our functions folder:

Hereβs what we will need to import into our emote.py file:
from highrise import * from highrise.models import * from highrise.webapi import * from highrise.models_webapi import *
Letβs proceed to our function definition, since weβre using the command handler for this example, your function name will be the command that you will use in the chat to call it, I will name mine as equip:
from highrise import * from highrise.webapi import * from highrise.models_webapi import * from highrise.models import * async def emote(self: BaseBot, user: User, message: str):
Finally, letβs implement the function and after it you will see a detailed step by step guide on how the code works:
from highrise import * from highrise.models import * from highrise.webapi import * from highrise.models_webapi import * async def emote(self: BaseBot, user: User, message: str) -> None: try: command, target, emote_id = message.split(" ") except: await self.highrise.chat("Invalid command format. Please use '/emote <target> <emote_id>.") return user = (await self.webapi.get_users(username=target)) if user.total == 0: await self.highrise.chat("Invalid target.") return user_id = user.users[0].user_id try: await self.highrise.send_emote(emote_id, user_id) except: await self.highrise.chat("Invalid emote.") return
Now letβs understand how the code works:
from highrise import *
,from highrise.models import *
,from highrise.webapi import *
, andfrom highrise.models_webapi import *
:- These lines import the necessary modules and classes required for interacting with the game's API, both for the main bot logic and for making API requests.
async def emote(self: BaseBot, user: User, message: str) -> None:
:- This is an asynchronous function called
emote
. - It takes four parameters:
self
(a reference to the instance of the class this method belongs to),user
(an object representing the user who sent the chat message), andmessage
(a string representing the chat message sent by the user).
try: command, target, emote_id = message.split(" ") except: await self.highrise.chat("Invalid command format. Please use '/emote <target> <emote_id>'.") return
:- The code uses a try-except block to split the
message
into three parts:command
,target
, andemote_id
. It expects the message to be in the format "/emote <target> <emote_id>". - If the split fails (likely due to an incorrect format), it means the user didn't provide the required three arguments.
- In that case, the function sends a message to the chat using
self.highrise.chat()
to inform the user about the invalid command format and then returns, ending the function.
user = (await self.webapi.get_users(username=target))
:- The code calls an asynchronous method
get_users(username=target)
onself.webapi
to fetch user data based on the specifiedtarget
username. - The result is awaited using
await
. - The method seems to return some kind of response object, and
user
appears to contain the user data.
if user.total == 0:
:- The code checks if the
total
attribute of theuser
object is 0, which would mean that no user was found with the specifiedtarget
username. - If no user is found, the function sends a message to the chat using
self.highrise.chat()
to inform the user about the invalid target and then returns, ending the function.
user_id = user.users[0].user_id
:- If a user is found with the specified
target
username, the code assigns theuser_id
of the first user in the response (user.users[0]
) to theuser_id
variable.
try: await self.highrise.send_emote(emote_id, user_id) except: await self.highrise.chat("Invalid emote.") return
:- The code uses another try-except block to attempt to send an emote to the specified target user using
self.highrise.send_emote(emote_id, user_id)
. - If the emote sending fails (likely due to an invalid
emote_id
or some other issue), the exception is caught. - In that case, the function sends a message to the chat using
self.highrise.chat()
to inform the user about the invalid emote and then returns, ending the function.
Β
Β