Get Started - Learn How To Make Your Bot!Code SnippetsGet methodsPost methodsWeb APIUseful linksList of all currently Free ItemsList of all currently Free EmotesHighrise Bot SDK Changelog
Concept
Want a easier way to remove an item from your bot’s outfit? You found it!
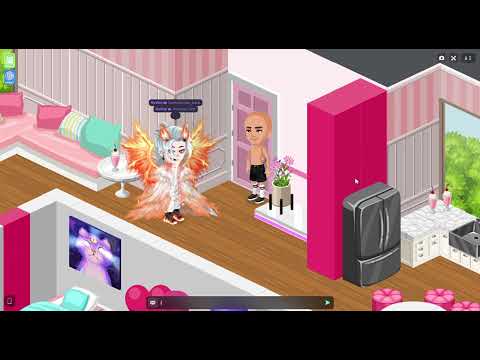
The code that we’re going to use today is very similar to the code at 👗 Equip Items.
Today we are gonna make a function to remove an item from your bot’s outfit using on_chat commands. To do that we will use the 📬 Command Handler, note that this use is optional, you can implement this function directly on your main code or directly on your on_chat method.
The command works by saying the command (remove), and the item category.
Categories
aura
bag
blush
body
dress
earrings
emote
eye
eyebrow
face_hair
fishing_rod
freckle
fullsuit
glasses
gloves
hair_back
hair_front
handbag
hat
jacket
lashes
mole
mouth
necklace
nose
pants
rod
shirt
shoes
shorts
skirt
sock
tattoo
watch
MAKE SURE TO READ HOW CHANGING THE OUTFIT WORKS ABOVE:
Methods/Functions used:
Required Libraries
- highrise-bot-sdk
Code
First of all, let’s create a new file called remove.py inside of our functions folder:

Here’s what we will need to import into our remove.py file:
from highrise import * from highrise.models import *
Let’s proceed to our function definition, since we’re using the command handler for this example, your function name will be the command that you will use in the chat to call it, I will name mine as color and I will create a list of valid categories:
from highrise import * from highrise.models import * categories = ["aura","bag","blush","body","dress","earrings","emote","eye","eyebrow","fishing_rod","freckle","fullsuit","glasses", "gloves","hair_back","hair_front","handbag","hat","jacket","lashes","mole","mouth","necklace","nose","rod","shirt","shoes", "shorts","skirt","sock","tattoo","watch"] async def remove(self: BaseBot, user: User, message: str):
Finally, let’s implement the function and after it you will see a detailed step by step guide on how the code works:
from highrise import * from highrise.models import * categories = ["aura","bag","blush","body","dress","earrings","emote","eye","eyebrow","fishing_rod","freckle","fullsuit","glasses", "gloves","hair_back","hair_front","handbag","hat","jacket","lashes","mole","mouth","necklace","nose","rod","shirt","shoes", "shorts","skirt","sock","tattoo","watch"] async def remove(self: BaseBot, user: User, message: str): parts = message.split(" ") if len(parts) != 2: await self.highrise.chat("Invalid command format. You need to specify the category.") return if parts[1] not in categories: await self.highrise.chat("Invalid category.") return category = parts[1].lower() outfit = (await self.highrise.get_my_outfit()).outfit for outfit_item in outfit: #the category of the item in an outfit can be found by the first string in the id before the "-" character item_category = outfit_item.id.split("-")[0][0:3] if item_category == category[0:3]: try: outfit.remove(outfit_item) except: await self.highrise.chat(f"The bot isn't using any item from the category '{category}'.") return response = await self.highrise.set_outfit(outfit) print(response)
Now let’s understand how the code works:
from highrise import *
andfrom highrise.models import *
:- These lines import the necessary modules and classes required for interacting with the game's API.
categories = [... list of category strings ...]
:- This is a list named
categories
, containing strings that represent different categories of items in the game. - Each string corresponds to a category, such as "aura", "bag", "blush", "body", etc.
async def remove(self: BaseBot, user: User, message: str):
- This is an asynchronous function named
remove
. - It takes four parameters:
self
(a reference to the instance of the class this method belongs to),user
(an object representing the user who sent the chat message), andmessage
(a string representing the chat message sent by the user).
parts = message.split(" ")
:- The function splits the
message
string into individual words using space as the separator and stores them in the listparts
.
if len(parts) != 2:
:- If there are not exactly two words in the
message
, it means the user has not specified the category correctly. - The function sends a message to the chat using
self.highrise.chat()
to inform the user about the invalid command format and then returns, ending the function.
if parts[1] not in categories:
:- The code checks if the second word in
parts
(i.e.,parts[1]
) does not match any of the categories listed incategories
. - If it doesn't match, it means the user entered an invalid category.
- The function sends a message to the chat using
self.highrise.chat()
to inform the user about the invalid category and then returns, ending the function.
category = parts[1]
:- After validating the category, the code assigns the category name to the variable
category
.
(await self.highrise.get_my_outfit()).outfit
:- The code calls an asynchronous method
get_my_outfit()
onself.highrise
to fetch the bot's outfit items. - The result is awaited using
await
. - The method seems to return some kind of response object, and
outfit
appears to be the actual list of items in the bot's outfit.
- The code then iterates through the outfit items to find the items of the specified
category
.
item_category = outfit_item.id.split("-")[0]
:- This line extracts the category of the current
outfit_item
by splitting the item's ID using the "-" character and taking the first part (before the "-").
if item_category == category:
:- If the category of the current
outfit_item
matches the specifiedcategory
, the code attempts to remove that item from the outfit usingoutfit.remove(outfit_item)
.
try: outfit.remove(outfit_item) except: await self.highrise.chat(f"The bot isn't using any item from the category '{category}'.") return
:- The code tries to remove the
outfit_item
from theoutfit
. If the item is not found in the outfit (e.g., it's already been removed or wasn't present in the first place), an exception is raised. - The code catches this exception and sends a message to the chat using
self.highrise.chat()
to inform the user that the bot isn't using any item from the specified category. - The function then returns, ending the execution.
await self.highrise.set_outfit(outfit)
:- After potentially removing the items from the specified category, the function calls an asynchronous method
set_outfit(outfit)
onself.highrise
. - It seems like this method is used to update the bot's outfit using the modified
outfit
list.